-
Notifications
You must be signed in to change notification settings - Fork 212
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
raise NotImplementedError("By default it is assumed that the data loader used for initialize " #1605
Comments
Greetings, @SiChuanJay! Dataloaders can return anything, and this output may be preprocessed in the rest of the training pipeline before actually ending up in model's from nncf.torch.initialization import PTInitializingDataLoader
class MyInitializingDataLoader(PTInitializingDataLoader):
def get_inputs(self, dataloader_output: Any) -> Tuple[Tuple, Dict]:
# your implementation - `dataloader_output` is what is returned by your dataloader,
# and you have to turn it into a (args, kwargs) tuple that is required by your model
# in this function, for instance, if your dataloader returns dictionaries where
# the input image is under key `"img"`, and your YOLOv8 model accepts the input
# images as 0-th `forward` positional arg, you would do:
return dataloader_output["img"], {}
def get_target(self, dataloader_output: Any) -> Any:
# and in this function you should extract the "ground truth" value from your
# dataloader, so, for instance, if your dataloader output is a dictionary where
# ground truth images are under a "gt" key, then here you would write:
return dataloader_output["gt"]
init_v8_dataloader = MyInitializingDataLoader(v8_dataloader)
# now you pass this wrapped object instead of your original dataloader into the `register_default_init_args`
nncf_config = register_default_init_args(nncf_config, init_v8_dataloader) |
@vshampor Thank you very much for your help. I need to quantify yolov8 by training-time method, but I am not familiar with both nncf and yolov8. With your help yesterday, the problem was solved, but errors were still reported during fine tuning, as shown below. I think it should be related to the v8 training mode. I tried to modify it, but it has not been solved. So can I ask for your help? Or can you provide relevant codes for yolov5 or yolov7 (training-time quantification). |
@SiChuanJay this problem has only been fixed recently in NNCF on the develop branch in #1600, so try installing NNCF from the Github's develop branch (or update your current NNCF state if you already did so) and try again. |
@vshampor Thank you for your help. I changed the version and solved the problem. But I had a new problem. When my batch=8, the error is the first picture. When my batch=4, the error is the second picture. So, I think there may be something wrong with the code I wrote. Could you please check it for me? Thank you very much! |
@SiChuanJay we can't check the code if we don't have it. Please provide complete info to reproduce this (the model/training code, datasets and command line parameters), or prepare a minimal reproducer if you don't want to share all of your data. |
This is my code, my aim is to quantify yolov8 using a training-time approach. And the yolov8n-seg.pt is from the official yolov8, it should download automatically. and the val dataset is from (http://images.cocodataset.org/zips/val2017.zip https://github.com/ultralytics/yolov5/releases/download/v1.0/coco2017labels-segments.zip) or you can look at this example(https://github.com/openvinotoolkit/openvino_notebooks/tree/main/notebooks/230-yolov8-optimization), which I downloaded using the link it provided. I really need your help, Thank you!
|
@vshampor Can you take a look at it? |
@SiChuanJay can i ask you if you solved the problem |
Hello, I ran into a problem with the training-time quantization of yolov8 using nncf, as shown below. I refer to the example link (https://github.com/openvinotoolkit/openvino_notebooks/tree/main/notebooks/302-pytorch-quantization-aware-training). My v8_dataloader is also of type DataLoader, but I got an error. Thanks very much!
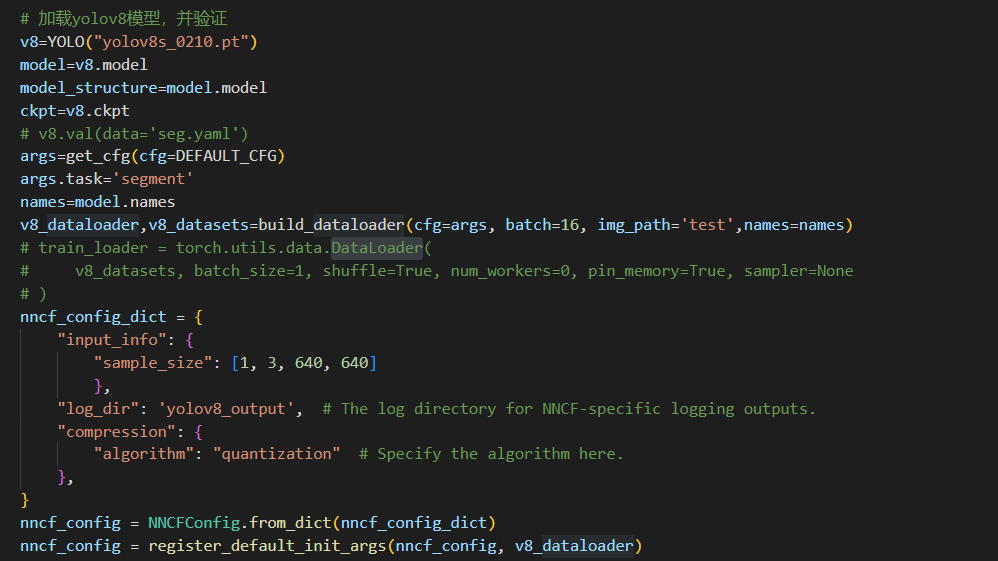

The text was updated successfully, but these errors were encountered: